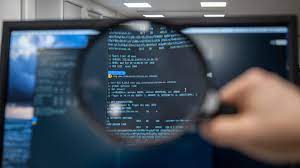
Flutter, Google’s open-source UI framework, is a popular choice among developers for building cross-platform mobile applications with a single codebase. However, like any other technology, Flutter can throw errors, and one of the common runtime errors developers often encounter is the NoSuchMethodError. In this blog post, we’ll dive into what this error means, why it happens in Flutter, and provide practical solutions with code examples to help you resolve it effectively.
Table of Contents
What is a NoSuchMethodError?
A NoSuchMethodError is a runtime error in Dart, the programming language used in Flutter, that occurs when you try to invoke a method on an object, but that object doesn’t have the method you’re trying to call. It’s important to understand the reasons behind this error to effectively deal with it.
#Why Does NoSuchMethodError Occur in Flutter?

Here are some common scenarios in Flutter where you might encounter a NoSuchMethodError:
Incorrect Method Name
The most frequent cause of this error is this. If you try to call a method with a name that doesn’t match any method defined for the object, Dart will raise a NoSuchMethodError. This often happens due to typographical errors in method names.
Null Objects
Attempting to call methods on null objects can lead to NoSuchMethodError because null objects do not have any methods. It’s essential to ensure that the objects you’re working with are not null before calling their methods.
Version Compatibility
Using code written for different versions of Dart can also trigger NoSuchMethodError. Ensure that your project and its dependencies are compatible with the Dart version you’re using.
How to Resolve NoSuchMethodError?
Now, let’s explore some strategies to resolve NoSuchMethodError in Flutter.
1. Check Method Names
Double-check the method names in your code. Ensure that the method you’re trying to call on an object exists and is spelled correctly. Dart is a case-sensitive language, so be mindful of letter casing.
2. Null Safety
Dart introduced null safety to help prevent issues related to null objects. Use the ? operator to safely call methods on potentially null objects. Here’s an example:
MyObject? obj = getNullableObject();
obj?.myMethod();
In this code, myMethod will only be called if obj is not null, preventing a NoSuchMethodError.
3. Dart Versions
Ensure that you’re using compatible Dart versions throughout your project. Check your pubspec.yaml file for dependencies and make sure they are up-to-date.
4. Use Try-Catch Blocks
To prevent your app from crashing when this error occurs, you can wrap the method call in a try-catch block. This way, you can gracefully handle the error and provide a fallback action.
try {
// Your method call here
} catch (e) {
// Handle the error gracefully
}
5. Utilize Optional Methods
In Dart and Flutter, you can make use of optional methods by using the ? operator. This allows you to call a method on an object even if it might be null, without triggering a NoSuchMethodError.
6. Debugging Tools
Flutter provides excellent debugging tools that can help you pinpoint the exact location where the NoSuchMethodError is occurring. Utilize these tools to identify and resolve the issue efficiently.
Code Example
Let’s illustrate these solutions with a practical code example. Consider the following Flutter code:
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Flutter NoSuchMethodError Example'),
),
body: Center(
child: ElevatedButton(
onPressed: () {
// Simulating a null object
String? message;
message?.toUpperCase(); // Using ? to prevent NoSuchMethodError
},
child: Text('Click Me'),
),
),
),
);
}
}
FAQs
1. What is a NoSuchMethodError in Flutter?
A NoSuchMethodError
in Flutter occurs when you try to call a method on an object that doesn’t have that method. It often happens due to method name mismatches or incorrect object types.
2. How can I avoid NoSuchMethodErrors in my Flutter code?
To avoid NoSuchMethodErrors
, always double-check method names, verify object types, and use try-catch blocks when necessary. Debugging tools in Flutter are also helpful for identifying and resolving such errors.
3. Can you provide an example of a NoSuchMethodError and how to fix it?
Certainly! Let’s say you have an object myObject
, and you try to call myObject.someMethod()
, but someMethod
doesn’t exist or is not defined for myObject
. To fix it, ensure that someMethod
is correctly defined for myObject
.
4. Is there any tool for debugging NoSuchMethodErrors in Flutter?
Yes, Flutter provides robust debugging tools like the Flutter DevTools and the Dart DevTools, which can help you identify and resolve NoSuchMethodErrors
efficiently.
5. Where can I learn more about Flutter error handling?
You can find more information about Flutter error handling and best practices in the official Flutter documentation and various online Flutter communities.
In this article, we’ve explored the intricacies of handling NoSuchMethodError
in Flutter, providing you with valuable insights and practical solutions to overcome this common challenge in your Flutter development journey.
You can also Read
Dart library ‘dart:html’ is not available on this platform.import ‘dart:html’
The named parameter ‘BackwardsCompatibility’ isn’t defined in flutter