When working with the FutureBuilder
widget in Flutter, it’s crucial to ensure that all required parameters are properly set. One common error that developers encounter is the message “The named parameter ‘future’ is required, but there’s no corresponding argument. Consider including the necessary reasoning. This problem usually arises because there is no valid Future object supplied to the future parameter, which makes the widget fail to create.
To understand this issue better, let’s delve into the nature of the FutureBuilder
widget. The FutureBuilder
is a powerful Flutter widget that helps in building UI components based on the latest snapshot of interaction with a Future
. It allows developers to create reactive and dynamic user interfaces that respond to asynchronous data fetching and processing. However, to leverage its capabilities, it’s vital to correctly set the future
parameter to a valid Future
object.
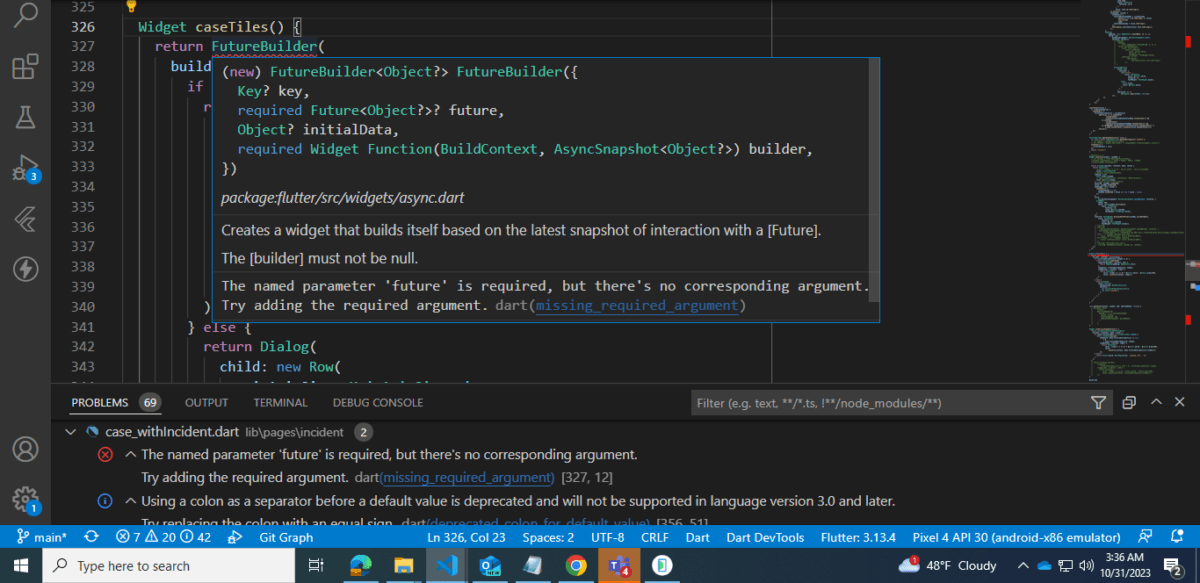
Let’s take a look at an example of how to use the FutureBuilder
widget and how to properly set the required parameters to avoid encountering this error.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: FutureBuilder(
// Error: 'future' parameter is missing
builder: (context, snapshot) {
// Handle snapshot data here
return Container();
},
),
),
),
);
}
}
In the above code snippet, we have omitted the future
parameter within the FutureBuilder
widget, leading to the error mentioned earlier. To resolve this issue, make sure to provide the future
parameter with a valid Future
object that fetches the required data asynchronously. For instance:
Future fetchData() {
// Simulated data fetching operation
return Future.delayed(Duration(seconds: 2), () => 'Data Fetched Successfully');
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: FutureBuilder(
future: fetchData(), // Assign a valid Future here
builder: (context, snapshot) {
// Handle snapshot data here
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator(); // Display a loading indicator
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return Text('Data: ${snapshot.data}');
}
},
),
),
),
);
}
}
Here is an example demonstrating how to assign the future
parameter of the FutureBuilder
widget by a variable of type Future
.
import 'package:flutter/material.dart';
Future<String> fetchData() {
// Simulated data fetching operation
return Future.delayed(Duration(seconds: 2), () => 'Data Fetched Successfully');
}
class MyApp extends StatelessWidget {
final Future<String> futureData = fetchData();
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Future Assigned by Variable Example'),
),
body: Center(
child: FutureBuilder(
future: futureData, // Assigning the future parameter using a variable of type Future
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator(); // Display a loading indicator
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return Text('Data: ${snapshot.data}');
}
},
),
),
),
);
}
}
In this example, the futureData
variable of type Future
is assigned the value returned by the fetchData
function. This futureData
variable is then used to assign the future
parameter of the FutureBuilder
widget. As a result, the FutureBuilder
starts fetching data as soon as it is built using the value stored in the futureData
variable. The UI will display a loading indicator while waiting for the future to complete and then show the data once it’s available.
Example : Loading Data from an API
import 'package:flutter/material.dart';
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<List<String>> fetchData() async {
final response = await http.get(Uri.parse('https://jsonplaceholder.typicode.com/posts'));
if (response.statusCode == 200) {
final data = json.decode(response.body);
return List<String>.from(data.map((item) => item['title']));
} else {
throw Exception('Failed to load data');
}
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('FutureBuilder Example'),
),
body: Center(
child: FutureBuilder<List<String>>(
future: fetchData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator(); // Display a loading indicator
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return ListView.builder(
itemCount: snapshot.data!.length,
itemBuilder: (context, index) {
return ListTile(
title: Text(snapshot.data![index]),
);
},
);
}
},
),
),
),
);
}
}
Example: Fetching and Displaying a List of User Data
import 'package:flutter/material.dart';
class User {
final String name;
final String email;
User(this.name, this.email);
}
Future<List<User>> fetchUsers() {
// Simulated data fetching operation
return Future.delayed(Duration(seconds: 2), () => [
User('John Doe', 'johndoe@example.com'),
User('Jane Smith', 'janesmith@example.com'),
User('Bob Johnson', 'bjohnson@example.com'),
]);
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('List of Users Example'),
),
body: Center(
child: FutureBuilder<List<User>>(
future: fetchUsers(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator(); // Display a loading indicator
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return ListView.builder(
itemCount: snapshot.data!.length,
itemBuilder: (context, index) {
final user = snapshot.data![index];
return ListTile(
title: Text(user.name),
subtitle: Text(user.email),
);
},
);
}
},
),
),
),
);
}
}
Here below is an example where the future
parameter is not assigned, resulting in a null
value.
import 'package:flutter/material.dart';
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('No Future Assigned Example'),
),
body: Center(
child: FutureBuilder(
future: null, // Assigning the future parameter as null
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return CircularProgressIndicator(); // Display a loading indicator
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
} else {
return Text('No data to display');
}
},
),
),
),
);
}
}
In this example, the future
parameter of the FutureBuilder
widget is deliberately set to null
. As a result, the FutureBuilder
enters the ConnectionState.waiting
state immediately and remains in that state since there is no actual future to wait for. It will never transition to the hasData
state or the hasError
state. Instead, it will display a loading indicator indefinitely.
This example serves to illustrate the importance of assigning a valid Future
object to the future
parameter of the FutureBuilder
widget to ensure proper handling of asynchronous data.
By providing a valid Future
object to the future
parameter, we ensure that the FutureBuilder
widget can correctly manage the asynchronous data and handle various states like loading, error, and data availability.
Remember, always check the documentation and ensure that all required parameters are properly set when using Flutter widgets to avoid encountering such errors.
You can also read: